Why Python?
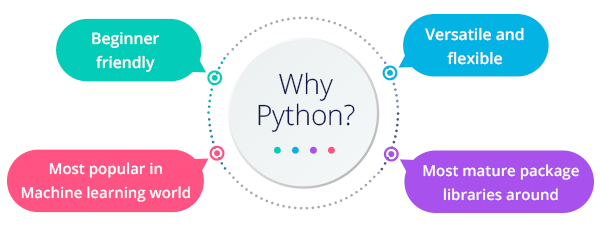
As I told about python in my previous blog that python is easy to learn, have vast libraries, less expensive and have many other features that's why many programmers and developers prefer python instead of the other languages. Here are quotes about python :
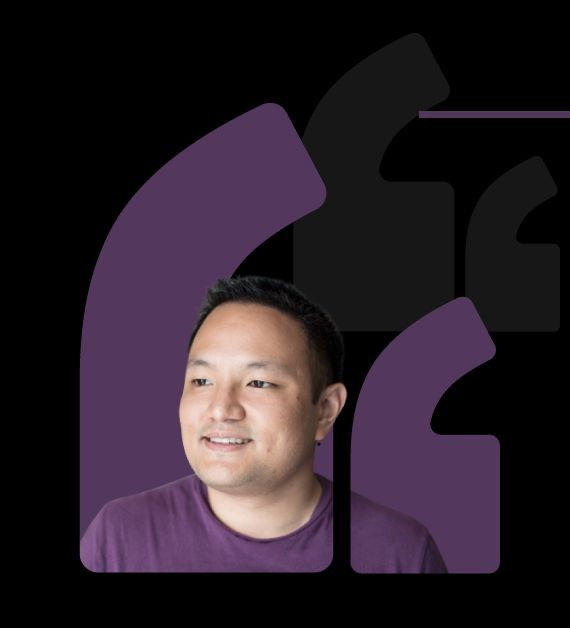
Python is fast enough for our site and allows us to produce maintainable features in record times, with a minimum of developers," said Cuong Do, Software Architect, YouTube.com.
"Python makes us extremely productive, and makes maintaining a large and rapidly evolving codebase relatively simple," said Mark Shuttleworth.
"I have the students learn Python in our undergraduate and graduate Semantic Web courses. Why? Because basically there's nothing else with the flexibility and as many web libraries," said Prof. James A. Hendler.
As you can see that many companies and professional in the field of computer science prefers python due its flexibility, many libraries and much more.
Get Started with Python:
Before starting with python you have to choose a perfect IDE, which will be best for you for example PyCharm, Visual Studio Code, Jupyter Notebook, etc. For beginner I personally prefer Jupyter Notebook but it is not mandatory and then after sometime you can install Visual Studio Code or PyCharm. For installing them go to the following youtube videos links:
Anaconda For Jupyter Notebook:
Linux:-
Windows:-
Visual Studio Code:
Linux:-
Windows:-
After installing and setting up Python you should begin :
Basic Python Syntax
Hello World Program:
The most basic thing of every language is printing "Hello World" using that language.
print("Hello World!")
Comments:
After hello world program, comments are the basics things moreover they are important in writing code. A good programmer always prefers writing comments which make his/her code much more understandable and perfect.
# This is a comment on its own line
var = "Hello, World!" # This is an inline comment
Own line comments are those comments in which there is no code but only comment while on the other hand inline comments are added with code to make that line understandable.
Variables:
Variables are those names which prefer to specific object or value. They store reference or pointer of that specific value. You can access that specific value which is assigned to that variable by calling variable name.
variable_name = variable_value
Above is the syntax for assigning value to variable.
However, there are some rules for naming varibles:
You can't start the name of variable with number or any special character.
You can start with uppercase letter but preferable is lower case letter.
Variable name should able to tell what is the purpose of that variable.
Avoid using single character variable name it can confuse later.
You can use underscore (_) in you varible name if it is long.
You can use inside the name of variable.
Variable name may be longer but try to avoid and write it simple and short name.
Keywords:
Like other programming language, python has some special words that are part of its syntax. These words are known us Keywords.
Here is a list of the Python keywords. Enter any keyword to get more help.
False class from or
None continue global pass
True def if raise
and del import return
as elif in try
assert else is while
async except lambda with
await finally nonlocal yield
break for not
To learn more about each keyword:
>>> help("keyword")
Built-in Data types:
As python is a dynamic language, it has some built in data type which automatically recognized by the IDE without mentioning and can easily change by the programmers.
Followings are the data types:
Numbers:
Numbers include all the real numbers in it. It contains both non decimal and decimal numbers. For non decimal numbers, type "int" is used while for decimal numbers, type "float" is used. For example:
>>>type(12)
output
<<<int
>>>type(23.5)
output
<<<float
>>> a = 345
>>> b = 99.9768
>>>type(a)
>>>type(b)
Output
<<<int
<<<float
In above example, you can see how show type of the numbers. We can also see that there is no need to mention type like other languages, Python IDE automatically recognize type of the variable.
Strings:
String is a data type of python in which sentences, character, etc can be store. We can write it in double or even in single quotation depends upon the programmer.
For example:
>>>type("Cyrus Programmer")
>>>type('Cyrus Programmer')
Output:
<<< str
str
>>> name = "Cyrus Programmer"
>>> name2 = 'Cyrus'
>>>type(name)
>>>type(name2)
Output:
<<< str
str
We can see that whether we use double quotation or single quotation it show it is string. String is like array which store values but it is mutable.
Boolean:
Boolean is another data type of Python. It store only True or False value in it or 0 or 1. This type is used when we apply some conditions in our program there many other uses of this type.
For example:
>>>type(True)
>>>type(False)
output:
<<<bool
<<<bool
>>>int(True)
<<< 1
>>>int(False)
<<< 0
In the above example, if we check the type of True or False it return bool and if we convert type of True or False it return 0 or 1 that's int values of True is 1 and False is 0.
Operators:
Operators represent operations such as addition, subtraction, multiplication, division, and so on. When you combines operators with numbers, they form expressions which python evaluate:
>>> # Addition
>>> 5 + 38
>>> # Subtraction
>>> 5 - 32
>>> # Multiplication>
>> 5 * 315
>>> # Division
>>> 6 / 31.6666666667
>>> # Floor division
>>> 5 // 31
>>> # Modulus (returns the remainder from division)
>>> 5 % 32
>>> # Power
>>> 5 ** 3125
These operators work with two operands and are commonly known as arithmetic operators. The operands can be numbers or variables that hold numbers.
Comments